Creating Neural Networks in JavaScript:
Quick-Start Guide
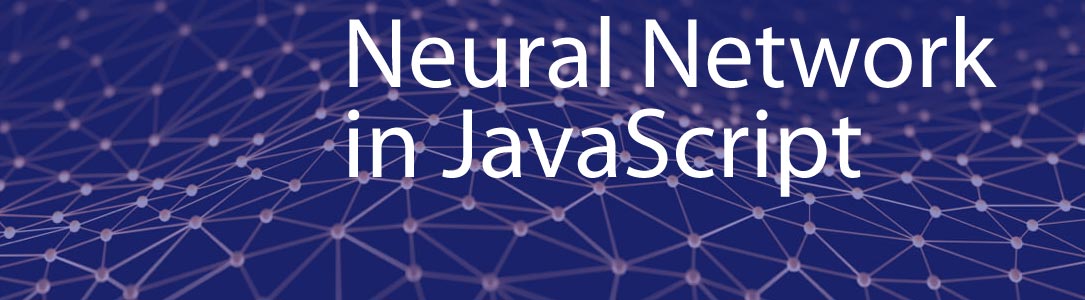
Neural networks and machine learning, a field of computer science that heavily relies on them to give computers the ability to learn without being explicitly programmed, seem to be everywhere these days.
Scientists want to use advanced neural networks to find energy materials, the Wall Street would like to train neural networks to manage hedge funds and pick stocks, and Google has been relying on neural networks to deliver highly accurate translations and transcriptions.
At the same time, powerful, consumer-grade hardware for machine learning is getting more affordable. For example, Nvidia has recently introduced its Titan V graphics card, which is targeted specifically at machine learning developers, who would like to create neural networks without paying for a special server to handle all the complex math operations involved in machine learning.
With the future of machine learning looking so bright and the necessary computational resources being so available, many JavaScript developers wonder what’s the easiest way how to create neural networks in JavaScript. If you count yourself among them, this article is for you.
What Are Neural Networks?
Neural networks, also known as artificial neural networks (ANNs), are algorithms inspired by the complex connections of neurons that constitute animal brains. What makes neural networks so special and so different from other algorithms is their ability to learn how to do various tasks without any task-specific programming.
But instead of just talking about neural networks, why not see how a neural network learns to perform a task in practice? And not just any task! FlappyLearning is a program written in JavaScript that automatically learns how to play Flappy Bird.
Speed up the game and watch how many generations it takes FlappyLearning to master Flappy Bird. Now, reload the page and do the same again. FlappyLearning will master Flappy Bird again, but it will either do so quicker or slower than before. That’s because neural networks are largely unpredictable in how they arrive at the desired result. In fact, Google’s AI translation tool even invented its own secret language, and so did Facebook’s robots.
Neural Networks Under the Hood
All neural networks, regardless of their complexity, consists of interconnected neurons. A neuron is a node which takes certain inputs and returns a certain output. Neural networks are comprised of multiple layers of neurons. Information in a neural network travels from the initial input layer through hidden layers, where the actual processing is done via a system of weighted connections, until it reaches the final output layer.
If you would like to learn more about how neural networks work under the hood, I highly recommend you watch video series from YouTube channel by 3blue1brown, which explains way more about neural networks than I can cover in this article.
To accomplish anything meaningful using the machine learning libraries described in the next section, you should also be proficient in JavaScript and jQuery. By far the best way how you can test your JavaScript skills is by obtaining a JavaScript certification from CancanIT, which is an online center for professional certification of web developers. They also offer a comprehensive jQuery certification, which is guaranteed to look great on your resume and help you build your first neural network.
Best JavaScript Machine Learning Libraries
JavaScript is a great language for learning about neural networks because there are many excellent libraries that make difficult things very simple.
Synaptic
Synaptic is an architecture-free neural network library for node.js and the web browser published under the MIT license. This is how easy it is to create a creature that learns how to explore the screen using Synaptic:
creature.js
1. var synaptic = require('synaptic'); 2. this.network = new synaptic.Architect.Perceptron(40, 25, 3);
world.js
1. creatures.forEach(function(creature) 2. { 3. // move 4. var input = []; 5. for (var i in creatures) 6. { 7. input.push(creatures[i].location.x); 8. input.push(creatures[i].location.y); 9. input.push(creatures[i].velocity.x); 10. input.push(creatures[i].velocity.y); 11. } 12. var output = creature.network.activate(input); 13. creature.moveTo(output); 14. 15. // learn 16. var learningRate = .3; 17. var target = [ 18. targetX(creature), 19. targetY(creature), 20. targetAngle(creature)]; 21. creature.network.propagate(learningRate, target); 22. });
To learn more about Synaptic, study the official documentation.
ConvNetJS
ConvNetJS is a JavaScript library for training neural networks in the browser. It was originally written by a PhD student at Stanford, but it is now maintained by an active community of machine learning enthusiasts from all over the world. The following example code is everything you need to get started with ConvNetJS:
1. <html> 2. <head> 3. <title>minimal demo</title> 4. 5. <!-- CSS goes here --> 6. <style> 7. body { 8. background-color: #FFF; /* example... */ 9. } 10. </style> 11. 12. <!-- import convnetjs library --> 13. <script src="convnet-min.js"></script> 14. 15. <!-- javascript goes here --> 16. <script type="text/javascript"> 17. 18. function periodic() { 19. var d = document.getElementById('egdiv'); 20. d.innerHTML = 'Random number: ' + Math.random() 21. } 22. 23. var net; // declared outside -> global variable in window scope 24. function start() { 25. // this gets executed on startup 26. //... 27. net = new convnetjs.Net(); 28. // ... 29. 30. // example of running something every 1 second 31. setInterval(periodic, 1000); 32. } 33. 34. </script> 35. </head> 36. 37. <body onload="start()"> 38. <div id="egdiv"></div> 39. </body> 40. </html>
deeplearn.js
deeplearn.js is a hardware-accelerated, open-source machine intelligence library for the web that promises to bring performant machine learning building blocks to all. The library was originally developed by the Google Brain PAIR team to build powerful interactive machine learning tools for the browser, but it’s now open to anyone. The following code is everything you need to create an image-recognition neural network using deeplearn.js:
JavaScript:
1. const catlmage = document.getElementById('cat'); 2. 3. const math = new dl.NDArrayMathGPU(); 4. // squeezenet is loaded from https://unpkg.com/deeplearn-squeezenet 5. const squeezeNet = new squeezenet.SqueezeNet(math); 6. await squeezeNet.load(); 7. 8. // Load the image into an NDArray from the HTMLImageElement. 9. const image = dl.Array3D.fromPixels(catImage); 10. 11. // Predict through SqueezeNet. 12. const logits = squeezeNet.predict(image); 13. 14. // Convert the logits to a map of class to probability of the class. 15. const topClassesToProbs = await squeezeNet.getTopKClasses(logits, 10); 16. for (const className in topClassesToProbs) [f| 17. console. log( 18. '${topClassesToProbs[className].toFixed(5)}: ${className}'); 19. }
HTML
1. <script src='https://unpkg.com/deeplearn-squeezenef></script> 2. <img id='cat' src='https://storage.googleapis.com/learnjs-data/images/cat.jpeg' crossorigin>
You may also be interested in: