What is Error Handling in PHP 7? Things you should know
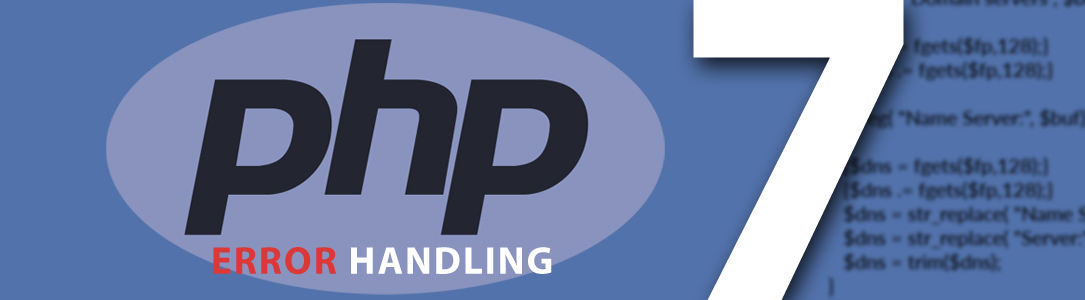
The developers are dealing with errors their entire lives and so the errors become an inevitable part of their code production process. A great coder is the one who ensures that the code is ready for handling all types of errors. An inbuilt error handling mechanism is provided that handles all exceptions and ensure that the overall code does not break or fail to execute because of an error in one component is considered as the best code in general.
In this article, we will have a look at the error handling procedure for PHP. Looking back at the older versions of PHP, it required to write special exceptional handling code by the developers to take care of the errors. While with the new release of PHP 7.x, it introduces several dedicated classes that take care of the process of exception handling. PHP has introduced two classes and discussed how these classes function in the real-time code process.
Throwable
It is the new reserved word introduced by PHP 7 which is also the name of a class from which two other classes namely Exception and Error are extended. The main function of this class is to catch errors if any, whether it is an exception or an error. Check out the given code snippet along with the parse error which is available in PHP 7.x.
1. <?php 2. try { 3. throw new Exception("This is an exception"); 4. } 5. catch(Throwable $e) { 6. echo $e->getMessage(); 7. }
1. <?php 2. try { 3. $result = eval("2*'7'"); 4. } 5. catch(Throwable $e){ 6. echo $e->getMessage(); 7. }
Error
It is another new reserved word in PHP 7 which refers to a new class introduced to handle several error exceptions including fatal errors and type errors. The Error class is important particularly in the context of upgrading your code to PHP 7.x. It results in Fatal Error failure in PHP 7.x if the code written in the previous versions has a custom class named Error and hence it requires to rename the class immediately.
The Error class has extended four subclasses directly from the class such as:
- Arithmetic Error
- Assertion Error
- Parse Error
- Type Error
Arithmetic Error
This subclass is used to catch the errors that occur during the execution of mathematical operations. Let’s understand it by an example, the use of intdiv() is for a number to get divided by 0 or -1 which throws an arithmetic error.
1. <?php 2. try { 3. var_dump(intdiv(PHP_INT_MIN, -1)); 4. } 5. catch(ArithmeticError $e){ 6. echo $e->getMessage(); 7. }
When you execute the above code, it displays the error because the shifted bit is negative. Also, another class, DivisionByZeroError also extends from Arithmetic Error subclass which has two different conditions. In the case of calculation of modulus of a number which is divided by 0, the error of DivisionByZeroError occurs and displays the Modulo by zero error message.
1. <?php 2. try { 3. $result = 5%0; 4. echo $result; 5. } 6. catch(DivisionByZeroError $e){ 7. echo $e->getMessage(); 8. }
After executing the given code, if you replace the modulus (%) with division (/), you get a warning by displaying the result either a 士INF or NAN. Another method of using the DivisionByZeroError exception in the following sample as given below. The intdiv() function is also used to throw DivisionByZeroError
1. <?php 2. try { 3. $result = is_finite(1.0 / 0); 4. if (in_array($result, [INF, NAN,-INF])) { 5. throw new DivisionByZeroError('Division by zero error'); 6. } 7. } 8. catch (DivisionByZeroError $e) { 9. echo $e->getMessage(); 10. }
Assertion Error
In the previous versions of PHP, you are required to create your own assertion exceptions handling functions for fatal errors in the case where assert_options() was used to bind custom functions which only occurs when an assertion is made through assert() gets failed. To overcome this, you have to configure the assert directives in PHP.ini file which has two options.
- Assert.exception - Its default value is 0 and merely generates a warning for the object rather than throwing an error. If the default value is changed to 1 then it throws an Assertion error which is caught by Throwable.
- Zend.assertions - In the production mode, its default value is -1 and the assertion code does not get generated. But when it is changed to 1 for development mode the code is generated and executed.
1. <?php 2. ini_set('assert.exception',1); 3. try{ 4. assert(2<1,"Two is not less than one"); 5. } 6. catch(AssertionError $ex) 7. { 8. echo $ex->getMessage(); 9. }
Parse Error
When you try to use eval() function to insert a new line into the code or use an external PHP file that has a syntax error, the parse error gets occurred. Let's consider a PHP file named index2.php:
1. <?php 2. $a = 4 3. $result = $a *5;
Next, we will call this file from another PHP file. When the given example is executed, it gets parse error instead of a fatal error as this subclass is very helpful in a number of scenarios.
1. <?php 2. try { 3. require "index3.php"; 4. } 5. catch(ParseError $e){ 6. echo $e->getMessage(); 7. }
Type Error
When the value of the data that you attempt to save is different from the value defined in the method or variable, type error subclass throws exceptions which are normally used with scalar type PHP 7 functions. Consider the example.
1. <?php 2. declare(strict_types=1); 3. function add(int $a, int $b) 4. { 5. return $a + $b; 6. } 7. try { 8. echo add("3","4"); 9. } 10. catch(TypeError $e){ 11. echo $e->getMessage(); 12. }
When the given code is executed, the TypeError exception is thrown. To overcome the error, you should remove the line declare(strict_types=1) from the code and execute it again in order to remove the exception.
Backward Compatibility
For PHP 7.x the backward compatibility is an important issue which inherits the fatal errors from the Error class. For the previous versions, where set_exception_handler() is used to set custom handlers and catch fatal errors. To avoid this, you should always remember to avoid custom handlers on PHP 7.x code and use the throwable class for custom exception catch and handling.
Multiple Exceptions
A single catch statement can be used to handle multiple exceptions which were introduced in PHP 7.1 as shown in the below code.
1. <?php 2. try { 3. // Some code... 4. } catch (ExceptionType1 | ExceptionType2 $e) { 5. // Code to handle the exception 6. } catch (\Exception $e) { 7. // ... 8. }
Wrap Up
From the previous version of PHP to 7.x, Exception handling is a tricky area that could cause problems in upgrading the code. It is crucial to remember that the process of exception catching and handling is greatly streamlined by the new classes.
You may also be interested in: